JavaScript
Introduction to React Higher order components
Introduction to React Higher order components
.png)
React HOCs are one of the most powerful features of React which allows extensive code reusability. Even if you are listening to this term for the first time, you will be surprised to know that you are already using HOCs in React if you are using libraries like react-router and redux.
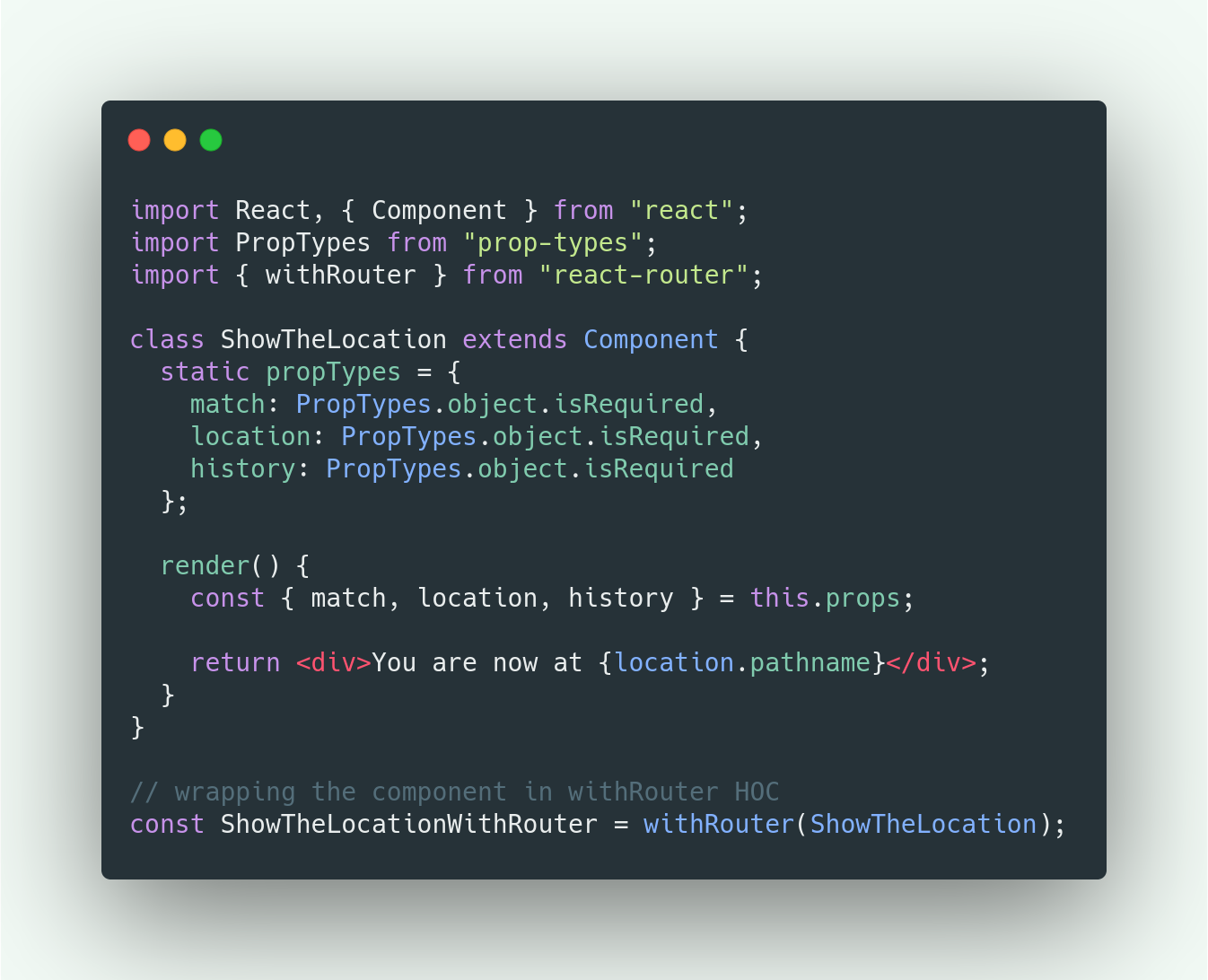
What does it do, you ask? withRouter allows the ShowTheLocation component to get access to the history object, the current location object, and the closest route match. withRouter will pass updated match, location, and history props to the wrapped component whenever it renders.
Another common example is connect when using the redux state management library. Connect function accepts parameters that allow it to connect a component to the global store in different ways. Examples can be, we don’t want to listen to the store just dispatch actions or we just want to listen to the store and don’t want to dispatch any actions or we want to do both. Connect returns an HOC that takes our component and returns a wrapped component with additional props it injects.
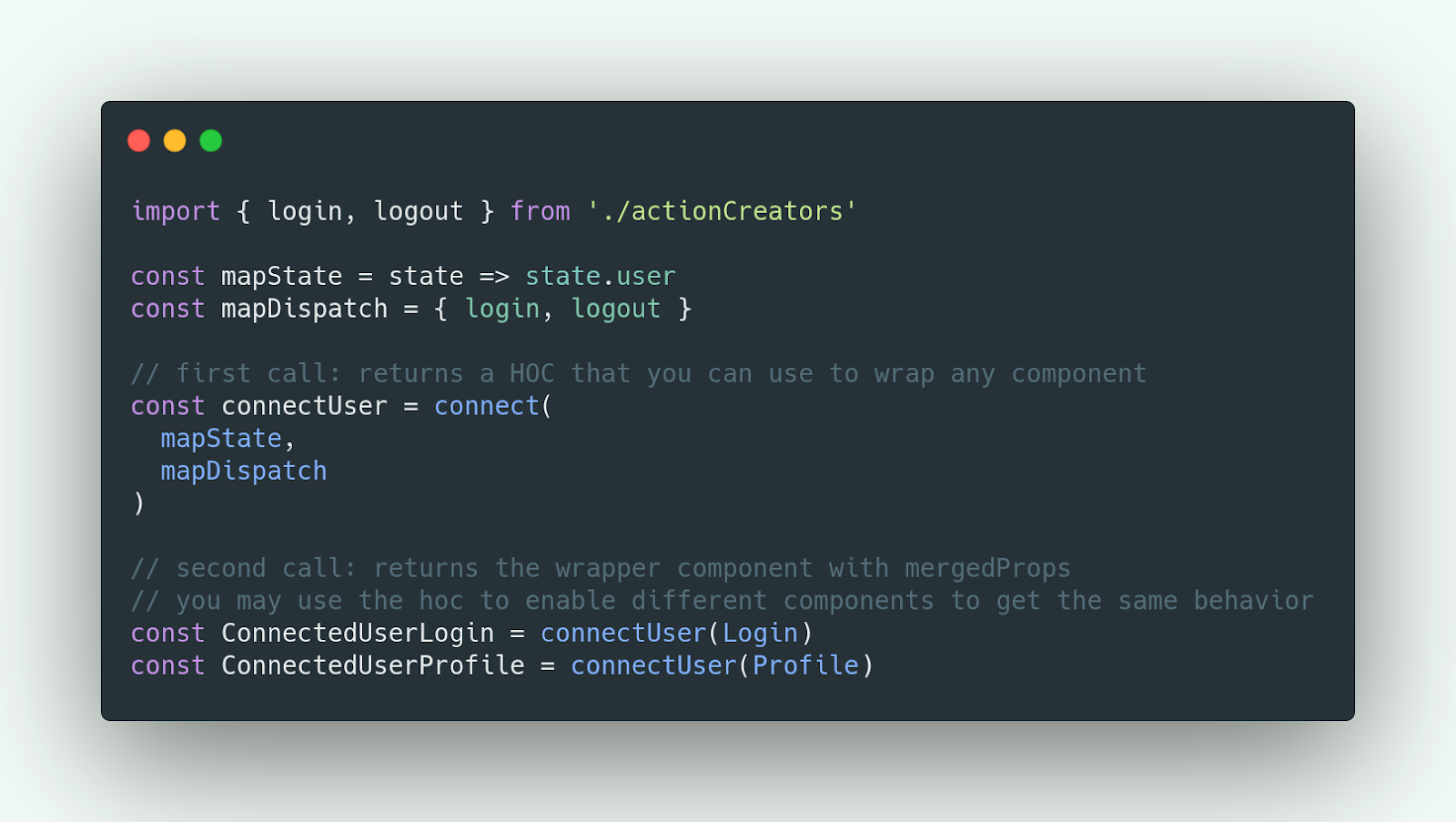
Let’s see what official docs say about HOCs.
A higher-order component (HOC) is an advanced technique in React for reusing component logic. HOCs are not part of the React API, per se. They are a pattern that emerges from React’s compositional nature.
Concretely, a higher-order component is a function that takes a component and returns a new component.
Now we will try to create a custom higher order component that will help us to understand it better. Remember, in higher order components we do not try to modify the wrapped component but try to add more functionality to it like additional props to the component.
Creating a basic HOC
Let’s create a basic higher order component UserAgent.jsx that will inject an additional prop userAgent to the wrapped component.
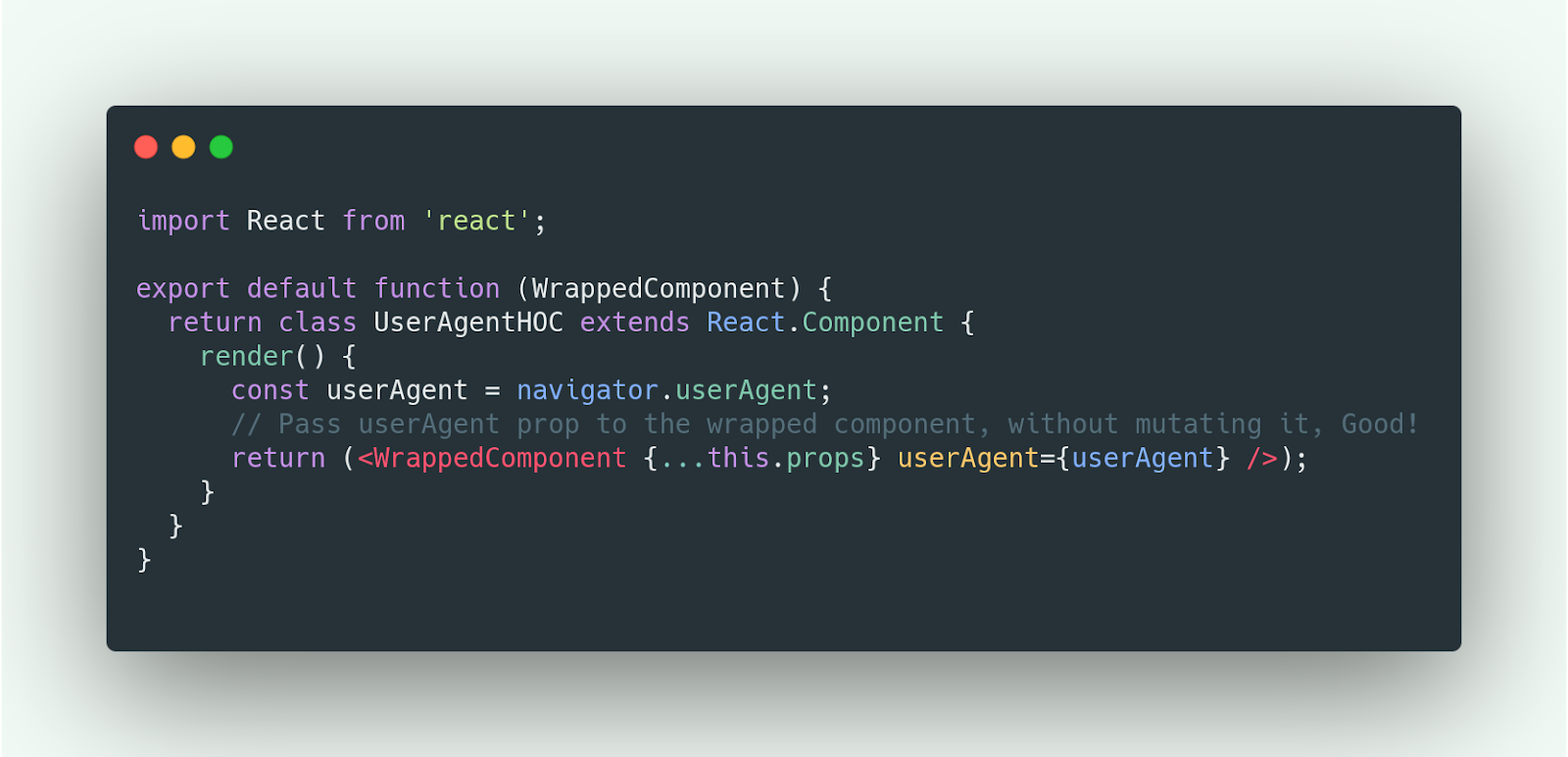
We are exporting default an anonymous function that returns a react component UserAgentHOC with additional prop userAgent. Now whenever we will wrap a component with this function, we can access userAgent prop.
How to use HOC
We have already created the higher order component. Now it’s time to test. We will create an example container MainContainer.jsx component that will be wrapped using this HOC.
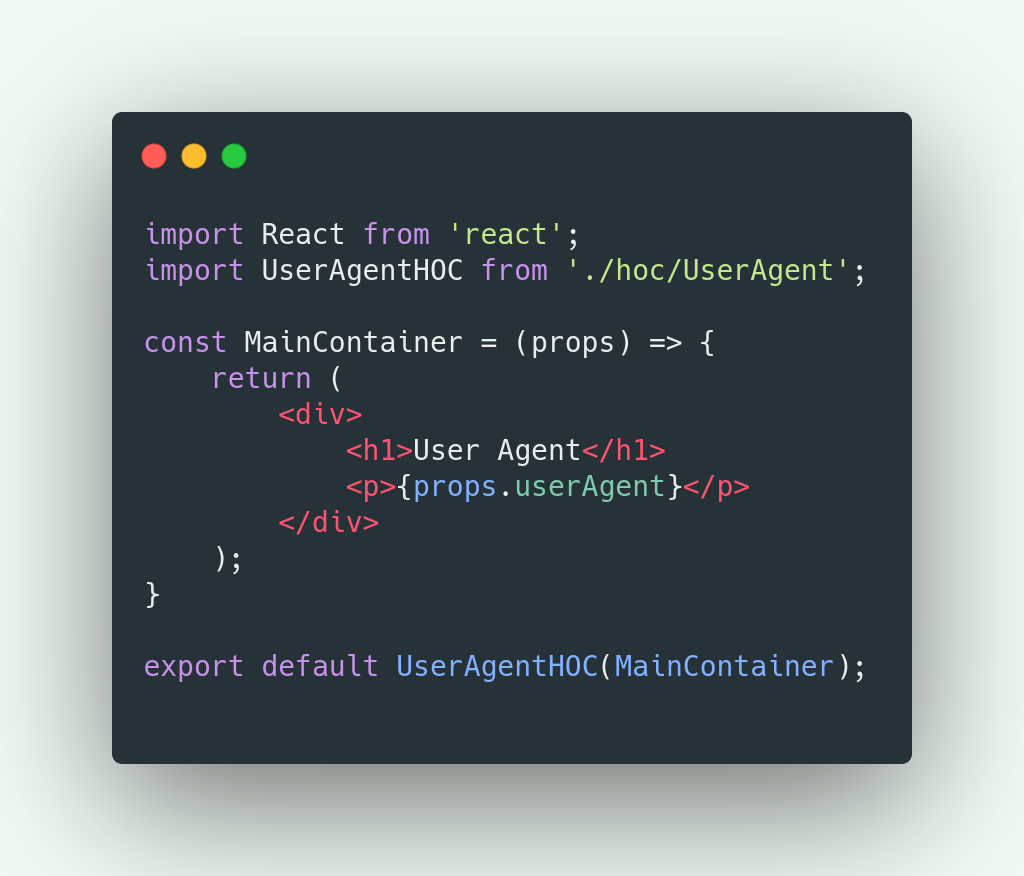
We’re wrapping up the MainContainer component with UserAgentHOC, so that it has access to userAgent prop and exporting it by default so that when we import the MainContainer component in any other component we have the enhanced component. Let’s import it in the App.js file.
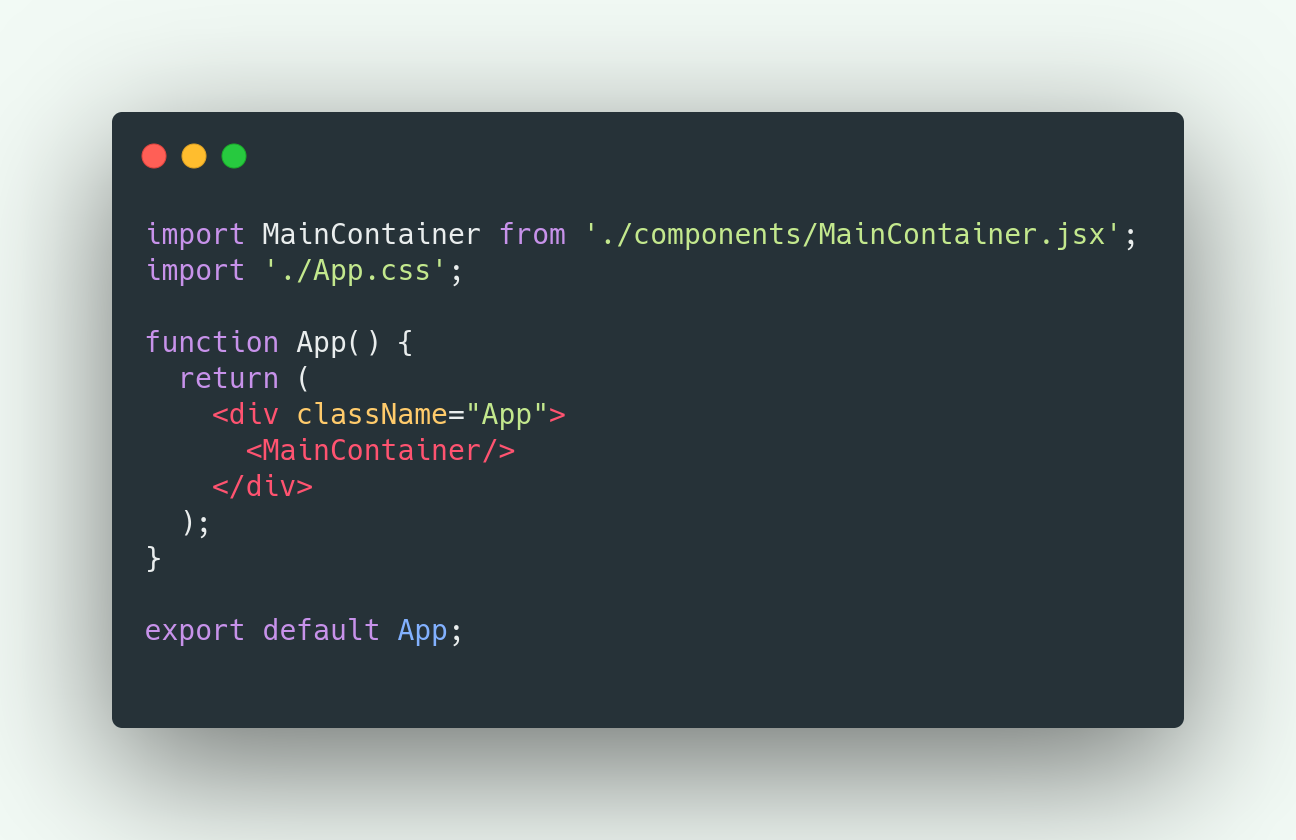
Now if we render this component, we will see the user agent string. So whenever we need a userAgent prop inside any component, we just wrap it with UserAgentHOC and we will have access to it.

Source code for this example can be found here.
Conclusion
Now we have a basic understanding about React HOCs in general and must be able to understand the other HOCs better which are mentioned earlier and how they work. This was a basic example to demonstrate how to get extensive code reusability in react with HOCs
With this concept in mind, we can now see which reusable props are required across different components and have a wrapper HOC to provide those.
Keep in mind that we do not modify the wrapped component, we just enhance it. Also an HOC is a pure function with no side effects, it just returns the enhanced component. With that being said, it’s time to take a look into official react docs talking more about HOCs.